Python Doppler Radar Viewer Documentation
Table of Contents
- Script Overview
- Dependencies
- Features
- Radar Locations
- Installation
- Usage
- Technical Details
- Supported Platforms
- Caveats
- Troubleshooting
- Doppler Radar Script
Script Overview
A Python script designed to download and display Doppler radar images based on user-selected locations. Currently supports Linux and Mac platforms.
Dependencies
- Python standard library
mpv
media player
Features
- Select radar locations from predefined list or manual input
- Download Doppler radar images for Netherlands, Germany, and United States
- Automatically cache and refresh radar images
- Interactive command-line interface
Radar Locations
The script supports the following radar locations:
- Netherlands (EU:NL:NL)
- Germany (EU:DE:DE)
- United States (NA:US:US)
Installation
No additional installation required beyond Python and mpv
.
Usage
- Run the script in a terminal
- Choose from the main menu:
- Show Doppler radar
- Change default radar location
- Exit
Example Workflow
Doppler Radar Script
1. Show Doppler radar
2. Change default radar
3. Exit
Select an option: 1
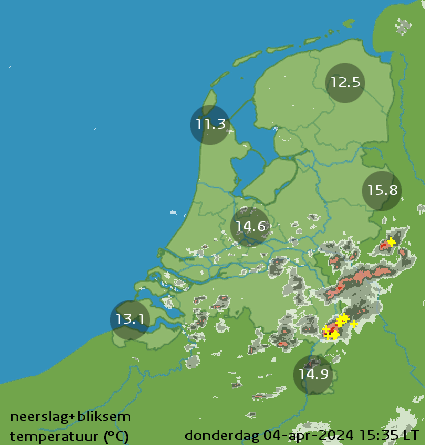
Technical Details
- Radar images are cached in
~/.cache/doppler.gif
- Radar location is stored in
~/.cache/radar
- Images are automatically refreshed every 10 minutes
Supported Platforms
- Linux
- macOS
Caveats
- Requires internet connection to download radar images
- Dependent on external radar service availability
Troubleshooting
- Ensure
mpv
is installed - Check internet connectivity
- Verify cache directory permissions
Doppler Radar Script
import os
import subprocess
import time
# Define constants
SECS = 600
RADAR_LOC = os.path.join(os.getenv('XDG_CACHE_HOME', os.path.expanduser('~/.cache')), 'radar')
DOPPLER = os.path.join(os.getenv('XDG_CACHE_HOME', os.path.expanduser('~/.cache')), 'doppler.gif')
def pickloc():
print("Available radars:")
print("1. EU:NL:NL (Netherlands)")
print("2. EU:DE:DE (Germany)")
print("3. NA:US:US (United States)")
choices = {
"1": "EU:NL:NL",
"2": "EU:DE:DE",
"3": "NA:US:US"
}
chosen = input("Select a radar by number (or enter manually in 'continent:country:radarcode' format): ")
if chosen in choices:
chosen = choices[chosen]
try:
continentcode, countrycode, radarcode = chosen.split(':')
with open(RADAR_LOC, 'w') as f:
f.write(f"{countrycode},{radarcode}\n")
except ValueError:
print("Invalid input format. Please try again.")
pickloc()
# Function to get Doppler
def getdoppler():
with open(RADAR_LOC, 'r') as f:
country, province = f.readline().strip().split(',')
print(f"Pulling most recent Doppler RADAR for {province}.")
if country == 'US':
subprocess.run(["curl", "-sL", f"https://radar.weather.gov/ridge/standard/{province}_loop.gif", "-o", DOPPLER])
elif country == 'DE':
province = province.lower()
subprocess.run(["curl", "-sL", f"https://www.dwd.de/DWD/wetter/radar/radfilm_{province}_akt.gif", "-o", DOPPLER])
elif country == 'NL':
subprocess.run(["curl", "-sL", "https://cdn.knmi.nl/knmi/map/general/weather-map.gif", "-o", DOPPLER])
# Function to show Doppler
def showdoppler():
subprocess.Popen(["mpv", "--no-osc", "--loop=inf", "--no-terminal", DOPPLER])
# Main function
def main():
while True:
print("\nDoppler Radar Script")
print("1. Show Doppler radar")
print("2. Change default radar")
print("3. Exit")
choice = input("Select an option: ")
if choice == '1':
if not os.path.isfile(RADAR_LOC):
print("No radar set. Please set a default radar first.")
pickloc()
if time.time() - os.path.getmtime(DOPPLER) > SECS:
getdoppler()
showdoppler()
elif choice == '2':
pickloc()
getdoppler()
elif choice == '3':
print("Exiting...")
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
Related
Software
Software